Monday, October 20, 2014
C++ Virtual Function
C++ Virtual Function
If there are member functions with same name in base class and derived class, virtual functions gives programmer capability to call member function of different class by a same function call depending upon different context. This feature in C++ programming is known as polymorphism which is one of the important feature of OOP.
If a base class and derived class has same function and if you write code to access that function using pointer of base class then, the function in the base class is executed even if, the object of derived class is referenced with that pointer variable. This can be demonstrated by an example.
#include <iostream>
using namespace std;
class B
{
public:
void display()
{ cout<<"Content of base class.\n"; }
};
class D : public B
{
public:
void display()
{ cout<<"Content of derived class.\n"; }
};
int main()
{
B *b;
D d;
b->display();
b = &d; /* Address of object d in pointer variable */
b->display();
return 0;
}
Note: An object(either normal or pointer) of derived class is type compatible with pointer to base class. So,
b = &d;
is allowed in above program.
Output
Content of base class. Content of base class.
In above program, even if the object of derived class d is put in pointer to base class,
display( )
of the base class is executed( member function of the class that matches the type of pointer ).Virtual Functions
If you want to execute the member function of derived class then, you can declare
display( )
in the base class virtual which makes that function existing in appearance only but, you can't call that function. In order to make a function virtual, you have to add keyword virtual in front of a function./* Example to demonstrate the working of virtual function in C++ programming. */
#include <iostream>
using namespace std;
class B
{
public:
virtual void display() /* Virtual function */
{ cout<<"Content of base class.\n"; }
};
class D1 : public B
{
public:
void display()
{ cout<<"Content of first derived class.\n"; }
};
class D2 : public B
{
public:
void display()
{ cout<<"Content of second derived class.\n"; }
};
int main()
{
B *b;
D1 d1;
D2 d2;
/* b->display(); // You cannot use this code here because the function of base class is virtual. */
b = &d1;
b->display(); /* calls display() of class derived D1 */
b = &d2;
b->display(); /* calls display() of class derived D2 */
return 0;
}
Output
Content of first derived class. Content of second derived class.
After the function of base class is made virtual, code
b->display( )
will call the display( )
of the derived class depending upon the content of pointer.
In this program,
display( )
function of two different classes are called with same code which is one of the example of polymorphism in C++ programming using virtual functions.C++ Abstract class and Pure virtual Function
In C++ programming, sometimes inheritance is used only for the better visualization of data and you do not need to create any object of base class. For example: If you want to calculate area of different objects like: circle and square then, you can inherit these classes from a shape because it helps to visualize the problem but, you do not need to create any object of shape. In such case, you can declare shape as a abstract class. If you try to create object of a abstract class, compiler shows error.
Declaration of a Abstract Class
If expression
=0
is added to a virtual function then, that function is becomes pure virtual function. Note that, adding =0
to virtual function does not assign value, it simply indicates the virtual function is a pure function. If a base class contains at least one virtual function then, that class is known as abstract class.Example to Demonstrate the Use of Abstract class
#include <iostream>
using namespace std;
class Shape /* Abstract class */
{
protected:
float l;
public:
void get_data() /* Note: this function is not virtual. */
{
cin>>l;
}
virtual float area() = 0; /* Pure virtual function */
};
class Square : public Shape
{
public:
float area()
{ return l*l; }
};
class Circle : public Shape
{
public:
float area()
{ return 3.14*l*l; }
};
int main()
{
Square s;
Circle c;
cout<<"Enter length to calculate area of a square: ";
s.get_data();
cout<<"Area of square: "<<s.area();
cout<<"\nEnter radius to calcuate area of a circle:";
c.get_data();
cout<<"Area of circle: "<<c.area();
return 0;
}
In this program, pure virtual function
virtual float area() = 0;
is defined inside class Shape, so this class is an abstract class and you cannot create object of class ShapeC++ Function Overriding
C++ Function Overriding
If base class and derived class have member functions with same name and arguments. If you create an object of derived class and write code to access that member function then, the member function in derived class is only invoked, i.e., the member function of derived class overrides the member function of base class. This feature in C++ programming is known as function overriding.
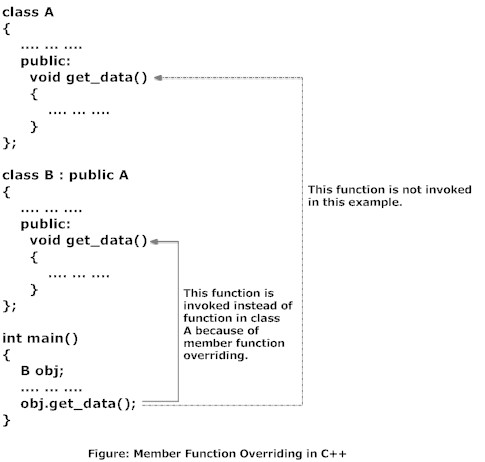
Accessing the Overridden Function in Base Class From Derived Class
To access the overridden function of base class from derived class, scope resolution operator ::. For example: If you want to access
get_data()
function of base class from derived class in above example then, the following statement is used in derived class.A::get_data; // Calling get_data() of class A.
It is because, if the name of class is not specified, the compiler thinks
get_data()
function is calling itself.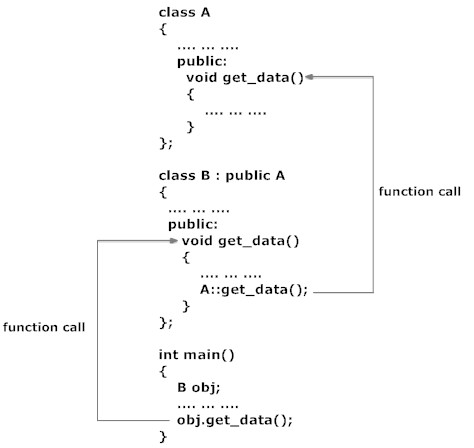
C++ Multilevel Inheritance and Multiple Inheritance
Levels of Inheritance
In C++ programming, a class be can derived from a derived class which is known as multilevel inhertiance. For example:
class A { .... ... .... }; class B : public A { .... ... .... }; class C : public B { .... ... .... };
In this example, class B is derived from class A and class C is derived from derived class B.
Example to Demonstrate the Multilevel Inheritance
#include <iostream>
using namespace std;
class A
{
public:
void display()
{
cout<<"Base class content.";
}
};
class B : public A
{
};
class C : public B
{
};
int main()
{
C c;
c.display();
return 0;
}
Output
Base class content.
Explanation of Program
In this program, class B is derived from A and C is derived from B. An object of class C is defined in
main( )
function. When the display( )
function is called, display( )
in class A is executed because there is no display( )
function in C and B. The program first looks for display( )
in class C first but, can't find it. Then, looks in B because C is derived from B. Again it can't find it. And finally looks it in A and executes the codes inside that function.
If there was
display( )
function in C too, then it would have override display( )
in A because ofmember function overriding.Multiple Inheritance in C++
In C++ programming, a class can be derived from more than one parents. For example: A classRectangle is derived from base classes Area and Circle.
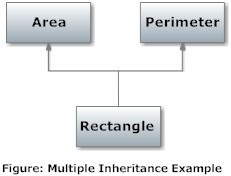
Source Code to Implement Multiple Inheritance in C++ Programming
This program calculates the area and perimeter of an rectangle but, to perform this program, multiple inheritance is used.
#include <iostream>
using namespace std;
class Area
{
public:
float area_calc(float l,float b)
{
return l*b;
}
};
class Perimeter
{
public:
float peri_calc(float l,float b)
{
return 2*(l+b);
}
};
/* Rectangle class is derived from classes Area and Perimeter. */
class Rectangle : private Area, private Perimeter
{
private:
float length, breadth;
public:
Rectangle() : length(0.0), breadth(0.0) { }
void get_data( )
{
cout<<"Enter length: ";
cin>>length;
cout<<"Enter breadth: ";
cin>>breadth;
}
float area_calc()
{
/* Calls area_calc() of class Area and returns it. */
return Area::area_calc(length,breadth);
}
float peri_calc()
{
/* Calls peri_calc() function of class Perimeter and returns it. */
return Perimeter::peri_calc(length,breadth);
}
};
int main()
{
Rectangle r;
r.get_data();
cout<<"Area = "<<r.area_calc();
cout<<"\nPerimeter = "<<r.peri_calc();
return 0;
}
Output
Enter length: 5.1 Enter breadth: 2.3 Area = 11.73 Perimeter = 14.8
Note: This program is intended to give you idea on how multiple inheritance works rather than the condition in which multiple inheritance is used.
Ambiguity in Multiple Inheritance
Multiple inheritance may be helpful in certain cases but, sometimes odd sort of problem encounters while using multiple inheritance. For example: Two base classes have functions with same name which is not overridden in derived class and if you write code to access that function using object of derived class, compiler shows error because, it cannot determine which function to call. Here is a code for this type of ambiguity in multiple inheritance
class base1
{
public:
void some_function( )
{ .... ... .... }
};
class base2
{
void some_function( )
{ .... ... .... }
};
class derived : public base1, public base2
{
};
int main()
{
derived obj;
/* Error because compiler can't figure out which function to call either same_function( ) of base1 or base2 .*/
obj.same_function( )
}
But, this problem can be solved easily using scope resolution function to specify which function to class either base1 or base2
int main() { obj.base1::same_function( ); /* Function of class base1 is called. */ obj.base2::same_function( ); /* Function of class base2 is called. */ }
C++ Inheritance
C++ Inheritance
Inheritance is one of the key feature of object-oriented programming including C++ which allows user to create a new class(derived class) from a existing class(base class). The derived class inherits all feature from a base class and it can have additional features of its own.

Concept of Inheritance in OOP
Suppose, you want to calculate either area, perimeter or diagonal length of a rectangle by taking data(length and breadth) from user. You can create three different objects( Area, Perimeter andDiagonal) and asks user to enter length and breadth in each object and calculate corresponding data. But, the better approach would be to create a additional object Rectangle to store value of length and breadth from user and derive objects Area, Perimeter and Diagonal from Rectanglebase class. It is because, all three objects Area, Perimeter and diagonal are related to objectRectangle and you don't need to ask user the input data from these three derived objects as this feature is included in base class.

Implementation of Inheritance in C++ Programming
class Rectangle { ... .. ... }; class Area : public Rectangle { ... .. ... }; class Perimeter : public Rectangle { .... .. ... };
In the above example, class Rectangle is a base class and classes Area and Perimeter are the derived from Rectangle. The derived class appears with the declaration of class followed by a colon, the keyword public and the name of base class from which it is derived.
Since, Area and Perimeter are derived from Rectangle, all data member and member function of base class Rectangle can be accessible from derived class.
Note: Keywords private and protected can be used in place of public while defining derived class(will be discussed later).
Source Code to Implement Inheritance in C++ Programming
This example calculates the area and perimeter a rectangle using the concept of inheritance.
/* C++ Program to calculate the area and perimeter of rectangles using concept of inheritance. */
#include <iostream>
using namespace std;
class Rectangle
{
protected:
float length, breadth;
public:
Rectangle(): length(0.0), breadth(0.0)
{
cout<<"Enter length: ";
cin>>length;
cout<<"Enter breadth: ";
cin>>breadth;
}
};
/* Area class is derived from base class Rectangle. */
class Area : public Rectangle
{
public:
float calc()
{
return length*breadth;
}
};
/* Perimeter class is derived from base class Rectangle. */
class Perimeter : public Rectangle
{
public:
float calc()
{
return 2*(length+breadth);
}
};
int main()
{
cout<<"Enter data for first rectangle to find area.\n";
Area a;
cout<<"Area = "<<a.calc()<<" square meter\n\n";
cout<<"Enter data for second rectangle to find perimeter.\n";
Perimeter p;
cout<<"\nPerimeter = "<<p.calc()<<" meter";
return 0;
}
Output
Enter data for first rectangle to find area. Enter length: 5 Enter breadth: 4 Area = 20 square meter Enter data for second rectangle to find perimeter. Enter length: 3 Enter breadth: 2 Area = 10 meter
Explanation of Program
In this program, classes Area and Perimeter are derived from class Rectangle. Thus, the object of derived class can access the public members of Rectangle. In this program, when objects of classArea and Perimeter are created, constructor in base class is automatically called. If there was public member function in base class then, those functions also would have been accessible for objects aand p.
Keyword protected
In this program, length and breadth in the base class are protected data members. These data members are accessible from the derived class but, not accessible from outside it. This maintains the feature of data hiding in C++ programming. If you defined length and breadth as private members then, those two data are not accessible to derived class and if defined as public members, it can be accessible from both derived class and from
main( )
function.Accessbility | private | protected | public |
---|---|---|---|
Accessible from own class ? | yes | yes | yes |
Accessible from dervied class ? | no | yes | yes |
Accessible outside dervied class ? | no | no | yes |
Member Function Overriding in Inheritance
Suppose, base class and derived class have member functions with same name and arguments. If you create an object of derived class and write code to access that member function then, the member function in derived class is only invoked, i.e., the member function of derived class overrides the member function of base class.
C++ Operator Overloading
C++ Operator Overloading
The meaning of operators are already defined and fixed for basic types like: int, float, double etc in C++ language. For example: If you want to add two integers then, + operator is used. But, for user-defined types(like: objects), you can define the meaning of operator, i.e, you can redefine the way that operator works. For example: If there are two objects of a class that contain string as its data member, you can use + operator to concatenate two strings. Suppose, instead of strings if that class contains integer data member, then you can use + operator to add integers. This feature in C++ programming that allows programmer to redefine the meaning of operator when they operate on class objects is known as operator overloading.
Why Operator overloading is used in C++ programming?
You can write any C++ program without the knowledge of operator overloading. But, operator operating are profoundly used by programmer to make a program clearer. For example: you can replace the code like:
calculation = add(mult(a,b),div(a,b));
with calculation = a*b+a/b;
which is more readable and easy to understand.How to overload operators in C++ programming?
To overload a operator, a operator function is defined inside a class as:
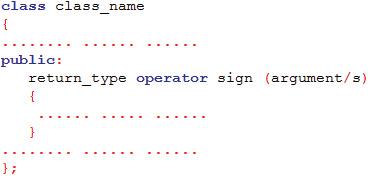
The return type comes first which is followed by keyword operator, followed by operator sign,i.e., the operator you want to overload like: +, <, ++ etc. and finally the arguments is passed. Then, inside the body of you want perform the task you want when this operator function is called.
This operator function is called when, the operator(sign) operates on the object of that class class_name.
Example of operator overloading in C++ Programming
/* Simple example to demonstrate the working of operator overloading*/
#include <iostream>
using namespace std;
class temp
{
private:
int count;
public:
temp():count(5){ }
void operator ++() {
count=count+1;
}
void Display() { cout<<"Count: "<<count; }
};
int main()
{
temp t;
++t; /* operator function void operator ++() is called */
t.Display();
return 0;
}
Output
Count: 6
Explanation
In this program, a operator function
void operator ++ ()
is defined(inside class temp), which is invoked when ++ operator operates on the object of type temp. This function will increase the value of count by 1.Things to remember while using Operator overloading in C++ language
- Operator overloading cannot be used to change the way operator works on built-in types. Operator overloading only allows to redefine the meaning of operator for user-defined types.
- There are two operators assignment operator(=) and address operator(&) which does not need to be overloaded. Because these two operators are already overloaded in C++ library. For example: Ifobj1 and obj2 are two objects of same class then, you can use code
obj1=obj2;
without overloading = operator. This code will copy the contents object of obj2 to obj1. Similarly, you can use address operator directly without overloading which will return the address of object in memory. - Operator overloading cannot change the precedence of operators and associativity of operators. But, if you want to change the order of evaluation, parenthesis should be used.
- Not all operators in C++ language can be overloaded. The operators that cannot be overloaded in C++ are ::(scope resolution), .(member selection), .*(member selection through pointer to function) and ?:(ternary operator).
Following best practice while using operator overloading
Operator overloading allows programmer to define operator the way they want but, there is a pitfall if operator overloading is not used properly. In above example, you have seen ++ operator operates on object to increase the value of count by 1. But, the value is increased by 1 because, we have used the code:
void operator ++() { count=count+1; }
If the code below was used instead, then the value of count will be decreased by 100 if ++ operates on object.
void operator ++() { count=count-100; }
But, it does not make any sense to decrease count by 100 when ++ operator is used. Instead of making code readable this makes code obscure and confusing. And, it is the job of the programmer to use operator overloading properly and in consistent manner.
Again, the above example is increase count by 1 is not complete. This program is incomplete in sense that, you cannot use code like:
t1=++t
It is because the return type of operator function is void. It is generally better to make operator work in similar way it works with basic types if possible.
Here, are the examples of operator overloading on different types of operators in C++ language in best possible ways:
- Increment and Decrement Operator Overloading
- Overloading of binary operator - to subtract complex number
Increment ++ and Decrement -- Operator Overloading in C++ Programming
In this tutorial, increment ++ and decrements -- operator are overloaded in best possible way, i.e., increase the value of a data member by 1 if ++ operator operates on an object and decrease value of data member by 1 if -- operator is used.
Increment Operator Overloading
/* C++ program to demonstrate the overloading of ++ operator. */
#include <iostream>
using namespace std;
class Check
{
private:
int i;
public:
Check(): i(0) { }
void operator ++()
{ ++i; }
void Display()
{ cout<<"i="<<i<<endl; }
};
int main()
{
Check obj;
/* Displays the value of data member i for object obj */
obj.Display();
/* Invokes operator function void operator ++( ) */
++obj;
/* Displays the value of data member i for object obj */
obj.Display();
return 0;
}
Output
i=0 i=1
Explanation
Initially when the object obj is declared, the value of data member i for object obj is 0( constructor initializes i to 0). When ++ operator is operated on obj, operator function
void operator++( )
is invoked which increases the value of data member i to 1.
This program is not complete in the sense that, you cannot used code:
obj1=++obj;
It is because the return type of operator function in above program is void. Here is the little modification of above program so that you can use code
obj1=++obj
./* C++ program to demonstrate the working of ++ operator overlading. */
#include <iostream>
using namespace std;
class Check
{
private:
int i;
public:
Check(): i(0) { }
Check operator ++() /* Notice, return type Check*/
{
Check temp; /* Temporary object check created */
++i; /* i increased by 1. */
temp.i=i; /* i of object temp is given same value as i */
return temp; /* Returning object temp */
}
void Display()
{ cout<<"i="<<i<<endl; }
};
int main()
{
Check obj, obj1;
obj.Display();
obj1.Display();
obj1=++obj;
obj.Display();
obj1.Display();
return 0;
}
Output
i=0 i=0 i=1 i=1
This program is similar to above program. The only difference is that, the return type of operator function is Check in this case which allows to use both codes
++obj;
obj1=++obj;
. It is because,temp returned from operator function is stored in object obj. Since, the return type of operator function is Check, you can also assign the value of obj to another object. Notice that, = (assignment operator) does not need to be overloaded because this operator is already overloaded in C++ library.Operator Overloading of Postfix Operator
Overloading of increment operator up to this point is only true if it is used in prefix form. This is the modification of above program to make this work both for prefix form and postfix form.
/* C++ program to demonstrate the working of ++ operator overlading. */
#include <iostream>
using namespace std;
class Check
{
private:
int i;
public:
Check(): i(0) { }
Check operator ++ ()
{
Check temp;
temp.i=++i;
return temp;
}
/* Notice int inside barcket which indicates postfix increment. */
Check operator ++ (int)
{
Check temp;
temp.i=i++;
return temp;
}
void Display()
{ cout<<"i="<<i<<endl; }
};
int main()
{
Check obj, obj1;
obj.Display();
obj1.Display();
obj1=++obj; /* Operator function is called then only value of obj is assigned to obj1. */
obj.Display();
obj1.Display();
obj1=obj++; /* Assigns value of obj to obj1++ then only operator function is called. */
obj.Display();
obj1.Display();
return 0;
}
Output
i=0 i=0 i=1 i=1 i=2 i=1
When increment operator is overloaded in prefix form;
Check operator ++ ()
is called but, when increment operator is overloaded in postfix form; Check operator ++ (int)
is invoked. Notice, theint inside bracket. This int gives information to the compiler that it is the postfix version of operator. Don't confuse this int doesn't indicate integer.Operator Overloading of Decrement -- Operator
Decrement operator can be overloaded in similar way as increment operator. Also, unary operators like: !, ~ etc can be overloaded in similar manner.
C++ Program to Subtract Complex Number Using Operator Overloading
In this tutorial, subtraction - operator is overloaded to perform subtraction of a complex number from another complex number. Since - is a binary operator( operator that operates on two operands ), one of the operands should be passed as argument to the operator function and the rest process is similar to the overloading of unary operators.
Binary Operator Overloading to Subtract Complex Number
/* C++ program to demonstrate the overloading of binary operator by subtracting one complex number from another. */
#include <iostream>
using namespace std;
class Complex
{
private:
float real;
float imag;
public:
Complex(): real(0), imag(0){ }
void input()
{
cout<<"Enter real and imaginary parts respectively: ";
cin>>real;
cin>>imag;
}
Complex operator - (Complex c2) /* Operator Function */
{
Complex temp;
temp.real=real-c2.real;
temp.imag=imag-c2.imag;
return temp;
}
void output()
{
if(imag<0)
cout<<"Output Complex number: "<<real<<imag<<"i";
else
cout<<"Output Complex number: "<<real<<"+"<<imag<<"i";
}
};
int main()
{
Complex c1, c2, result;
cout<<"Enter first complex number:\n";
c1.input();
cout<<"Enter second complex number:\n";
c2.input();
/* In case of operator overloading of binary operators in C++ programming, the object on right hand side of operator is always assumed as argument by compiler. */
result=c1-c2; /* c2 is furnised as an argument to the operator function. */
result.output();
return 0;
}
Explanation
In this program, three objects of type Complex is created and user is asked to enter the real and imaginary parts for two complex numbers which is stored in objects
c1
and c2
. Then statementresult=c1-c2
is executed. This statement invokes the operator function Complex operator - (Complex c2)
. When result=c1-c2
is executed, c2
is passed as argument to the operator function.In case of operator overloading of binary operators in C++ programming, the object on right hand side of operator is always assumed as argument by compiler. Then, this function returns the resultant complex number(object) to main() function and then, it is displayed.
Though, this tutorial contains the overloading of - operators, binary operators in C++ programming like: +, *, <, += etc. can be overloaded in similar manner.
Subscribe to:
Posts (Atom)